Guardrails AI
What is Guardrails?
Guardrails is a Python framework that helps build reliable AI applications by performing two key functions:
- Guardrails runs Input/Output Guards in your application that detect, quantify and mitigate the presence of specific types of risks. To look at the full suite of risks, check out Guardrails Hub.
- Guardrails help you generate structured data from LLMs.
Guardrails Hub
Guardrails Hub is a collection of pre-built measures of specific types of risks (called 'validators'). Multiple validators can be combined together into Input and Output Guards that intercept the inputs and outputs of LLMs. Visit Guardrails Hub to see the full list of validators and their documentation.
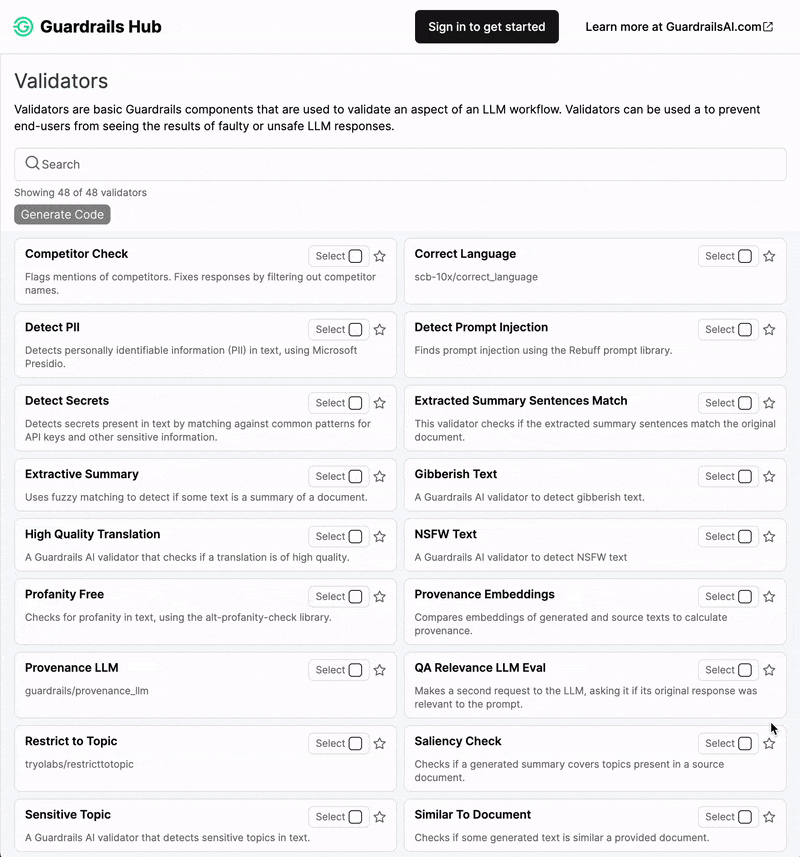